Decoupling your (event) system
Matthias Noback
About interface segregation, dependency inversion and package stability
You are creating a nice reusable package. Inside the package you want to use events to allow others to hook into your own code. You look at several event managers that are available. Since you are somewhat familiar with the Symfony EventDispatcher component already, you decide to add it to your package’s composer.json
:
{
"name": "my/package"
"require": {
"symfony/event-dispatcher": "~2.5"
}
}
Your dependency graph now looks like this:
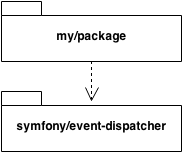
Introducing this dependency is not without any problem: everybody who uses my/package
in their project will also pull in the symfony/event-dispatcher
package, meaning they will now have yet another event dispatcher available in their project (a Laravel one, a Doctrine one, a Symfony one, etc.). This doesn’t make sense, especially because event dispatchers all do (or can do) more or less the same thing.
Though this may be a minor inconvenience to most developers, having just this one extra dependency may cause some more serious problems when Composer tries to resolve version constraints. Maybe a project or one of its dependencies already has a dependency on symfony/event-dispatcher
, but with version constraint >=2.3,<2.5
…
Some drawbacks of the Symfony EventDispatcher
On top of these usability issues, when it comes to design principles, depending on a concrete library like the Symfony EventDispatcher is not such a good choice either. The EventDispatcherInterface
which you will likely use in your code is quite bloated:
namespace Symfony\Component\EventDispatcher;
interface EventDispatcherInterface
{
public function dispatch($eventName, Event $event = null);
public function addListener($eventName, $listener, $priority = 0);
public function addSubscriber(EventSubscriberInterface $subscriber);
public function removeListener($eventName, $listener);
public function removeSubscriber(EventSubscriberInterface $subscriber);
public function getListeners($eventName = null);
public function hasListeners($eventName = null);
}
The interface basically violates the Interface Segregation Principle, which means that it serves too many different types of clients: most clients will only use the dispatch()
method to actually dispatch an event, and other clients will only use addListener()
and addSubscriber()
. The remaining methods are probably not even used by any of the clients, or only by clients that help you to debug your application (a quick search in the Symfony code-base confirms this suspicion).
Personally, I think it’s not really nice that events need to be objects extending the Event
class. I understand why Symfony does it (it’s basically because that class has the stopPropagation()
and isPropagationStopped()
methods which enables event listeners to stop the dispatcher from notifying the remaining listeners. I’ve never liked that, nor wanted it to happen in my code. Just like the original Observer pattern prescribes, all listeners (observers) should be able to respond to the current situation.
I also don’t like the fact that the same event class can be used for different events (differentiated by just their name, which is the first argument of the dispatch()
method). I prefer each type of event to have its own class. Therefore, to me it would make sense to pass just an event object to the dispatch()
method, allowing it to return its own name, which will always be the same anyway. The name returned by the event itself can then be used to determine which listeners need to be notified.
According to these objections to the design of the Symfony EventDispatcher classes, we’d be better off with the following clean interfaces:
namespace My\Package;
interface Event
{
public function getName();
}
interface EventDispatcher
{
public function dispatch(Event $event);
}
It would be really great to be able to just use these two interface in my/package
!
Symfony EventDispatcher: the good parts
Still, we also want to use the Symfony EventDispatcher. Above all we are familiar with it, but it also has some nice options, for example lazy-loading listeners, and when used in a Symfony application it offers an easy way to hook up listener services using service tags.
Introducing the Adapter
So we want to use our own event dispatching API, defined by the interfaces described above, but we also want to use the Symfony EventDispatcher which has a different public API. The solution is to create an adapter which bridges the gap between the two interfaces (following the famous Adapter pattern). In our case:
use My\Package\Event;
use My\Package\EventDispatcher;
use Symfony\Component\EventDispatcher\EventDispatcherInterface;
class SymfonyEventDispatcher implements EventDispatcher
{
private $eventDispatcher;
public function __construct(EventDispatcherInterface $eventDispatcher)
{
$this->eventDispatcher = $eventDispatcher;
}
public function dispatch(Event $event)
{
// call the relevant listeners registered to the Symfony event dispatchers
...
}
}
It can be a bit tricky to follow what’s going on because of all the names that are so much alike. But as you can see, a Symfony event dispatcher gets injected as a constructor argument into the adapter class. When the dispatch()
method gets called, the adapter forwards the call to the Symfony event dispatcher.
Well, it’s not very straight-forward to just forward the dispatch()
call to the Symfony event dispatcher, since as we saw earlier that its method signature looks like this:
namespace Symfony\Component\EventDispatcher;
interface EventDispatcherInterface
{
public function dispatch($eventName, Event $event = null);
...
}
And unfortunately we don’t have an object of type Symfony\Component\Event
lying around, and we don’t want one either, since that would totally reintroduce coupling to the Symfony component again.
Luckily the interface has another method we can use to bridge the gap: getListeners()
, which means we can fetch the listeners ourselves and notify them manually:
class SymfonyEventDispatcher implements EventDispatcher
{
...
public function dispatch(Event $event)
{
$listeners = $this->eventDispatcher->getListeners($event->getName());
foreach ($listeners as $listener) {
call_user_func($listener, $event);
}
}
}
We have now avoided the use of the Symfony Event
class entirely. Inside our package we only need to use our own EventDispatcher
interface and Event
interface. This means we can remove the dependency on the symfony/event-dispatcher
package altogether… almost!
The adapter class needs to be in its own package, to be able to fully decouple our package from symfony/event-dispatcher
. Depending on what other (Symfony) components we are going to decouple from, we might call the adapter package my/package-symfony-bridge
, or my/package-symfony-event-dispatcher
, etc.
The resulting package dependency graph is quite beautiful:
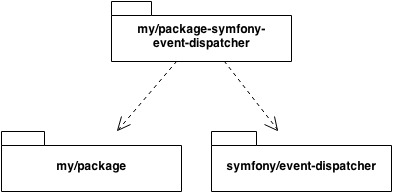
If you’ve read about package design principles before, you will know that this is a really nice constellation of packages because my/package
does not depend on symfony/event-dispatcher
anymore. In fact, it doesn’t depend on anything anymore. We call such a package an independent package. At the same time, there are only packages depending on it, which makes my/package
responsible. Being independent and responsible is what makes a package stable.
Also I’d like to point out that basically we have just applied an old and well-known design principle here: the Dependency inversion principle: we inverted the dependency direction. Instead of directly depending on a class (or in this case an interface) from another package, we defined our own interface. Then we created an adapter which enabled us to bridge the gap between our interface and the external interface.
Conclusion
There is one important conclusion to be mentioned here:
You don’t need any dependencies at all.
In theory, your package would never need to depend on another package. Instead, it could define all kinds of interfaces itself and let adapter packages implement them. This would automatically make your package highly stable. It also makes it extremely reusable, because a user only needs to implement those interfaces specifically for the framework they use (if you haven’t already done it for them!).
I’d like to mention the Aura packages here, which are designed in this way: no dependencies at all. It appears many people find this awkward and unnecessary, but given the approach described in this article, it should be more clear how such a thing is possible and even desirable.
If you’d like to know more about this subject: I’m writing a book called Principles of PHP Package Design. You can buy it now and get all future updates (meaning: new chapters) for free.